Create A Quiz Program In Java
Here you will get the online quiz system project in JSP (Java). Few days back my college organized a tech fest. There was a coding competition event in that fest. Total hands-on programming experience. All core Java object-oriented concepts covered. Every concept explained with the help of a program. Mini-project at the end which covers a lot of object oriented concepts. Requires 2-3 hours of your time. I believe you will enjoy this course and make the most of it.
A unit test in Java gives the developer the opportunity to test an object. For example, you might want to verify that a Person object contains the expected person name or perhaps the correct email address. In addition, you may want to verify that a property is not null.
The most common way to write unit tests in Java is to use JUnit, a free, open source unit-testing Java application. To learn how to write a unit test in Java, follow these seven steps.
- First, you need to download JUnit 4.8.2. In your browser, go to this page on github.com and click junit.jar. Another web page will be displayed.
- Click the jar option for junit 4.8.2. This action will start the download of
junit-4.8.2.jar
to your computer. - Now you will write the program that contains the JUnit tests. Open your text editor and type in the following statements:
The@BeforeClass
annotation marks a method that will be called by JUnit one time before the tests are performed. The@AfterClass
annotation marks a method that will be called once after the tests have been run. The@Test
annotations mark the tests that will be executed by JUnit. Two tests are included in the program. The first test will verify that the first name has been successfully set to 'Stephen'. This test will succeed at runtime. The second test will assert that the last name is not null. This test will fail at runtime because although the Person object has been instantiated, the last name has not been set. - Save your file as
WriteAUnitTest.java
. - Copy
junit-4.8.2.jar
to the directory that contains your Java program. - Open a command prompt and navigate to the directory containing your Java program. Then type in the command to compile the source using the classpath compiler option and hit Enter.
- Type in the command to run the JUnit test runner using your program and hit Enter.
The output indicates one test failed with anAssertionError
exception. The name of the failing method and the program name are displayed in the output.
See the Pen Simple JavaScript Quiz by Yaphi (@yaphi1) on CodePen.
The point of this tutorial is to make the simplest possible JavaScript quiz without any external code needed.
We'll also avoid animations, excessive styles, and anything else that will distract from the JavaScript quiz.
A Quick Note Before You Start:
This tutorial assumes a basic understanding of JavaScript. If you're not there yet, I've put together a JavaScript road map to get you comfortable with practical concepts quickly.
Step 1: Set up the structure
First, we'll create divs to hold our quiz and our results.
Then we'll put in a submit button.
Here's the HTML:
Next, we'll create a function to generate a quiz.
Your function will need these inputs:
- The quiz questions
- A place to put the quiz
- A place for the results
- A submit button
And if you put those things in, the function should spit out a fully-formed quiz.
The JavaScript structure:
If you look closely at the JavaScript structure, you'll see that our generateQuiz
function contains helper functions to show the quiz, accept submissions, and show the results.
Step 2: Show the questions
First we'll need the questions, so put this in your JavaScript:
Next we'll need a way to show our questions.
For this, we'll fill out our showQuestions
function.
The general idea:
For each question, show the question along with all of its answer choices. Read through the comments in this code to see how it works.
Once that's ready, you can run the function like this:
Note that in this line, the questions
and quizContainer
values will come from your generateQuiz
function.
The nice part about our code is that it works for any number of questions or answer choices you might have in your JavaScript quiz.
Step 3: On submit, show the results
We'll need to fill out our showResults
function to show the results of our quiz.
Here's how our JavaScript logic will look:
- For each question, find the selected answer
- If the answer is correct, respond accordingly
- If the answer is wrong, respond accordingly
- Show the number of correct answers out of the total
And here's the JavaScript to show the results of our quiz:
In the line that says '//find selected answer', we did a little trick. We used the operator, which means 'or' to basically say 'Give us the selected answer OR if there's not one, then just give us an empty object.' That way, trying to get the
.value
will give us undefined
instead of causing an error.
Laser Engraving Software free download - Canon LASER SHOT LBP-1120, Canon LASER SHOT LBP-1210, Bluetooth Software Ver.6.0.1.4900.zip, and many more programs. GRBL 1 1H download package. Laser for engraver software Engraver master setup. Other useful software tools. G-code sender zip (for Win64/32 and MacOS) InkScape software installation package Inkscape active directory RepetierHost installation package. Last stable version: latest All versions: github.com/arkypita/LaserGRBL Also source code of LaserGRBL is available under GPLv3 license. LaserGRBL is free. EzGraver is a free open source laser engraving software for Windows. Through this software, you can instruct various types of laser engraving machines to engrave a predefined design over a selected material. To import predefined designs, you can import images of various formats like BMP, GIF, ICO, JPG, PNG, TIFF, and more. Adjustable 1000mW laser head, convenient to adjust, ensure engraving with good quality and high efficiency. The engraving auxiliary fan is designed for heat dissipation and blow engraving smoke away quickly. Advanced software helps to edit picture and monitor the engraving process. Supercarver laser engraver software download.
That way, the quiz won't break if someone skips a question.
Show quiz results on submit
The next step is to show quiz results when someone clicks the submit button.
The following JavaScript will make that happen:
Note that the submitButton
variable comes from our original generateQuiz
function as one of the parameters.
Step 4: Put it all together
Now that you have the pieces, you can generate your very own JavaScript quiz.
You have the questions in the myQuestions
variable from Step 2. Now you need to let your JavaScript know which HTML elements to use for the quiz, the results, and the submit button.
Now that everything's in place, you can generate your JavaScript quiz!
Congrats!
Bonus: The fun part
Now that you have the basic idea of how to make a JavaScript quiz, you can try styling the elements however you want.
Sample CSS used in demo:
Create A Quiz Program In Javascript
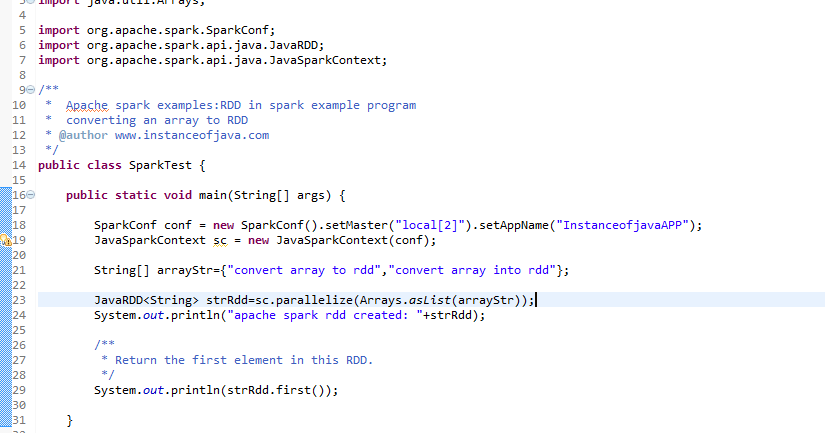
Create A Quiz Program In Java Programming
Feel free to change whatever you want!